- June 9, 2023
- nschool
- 0
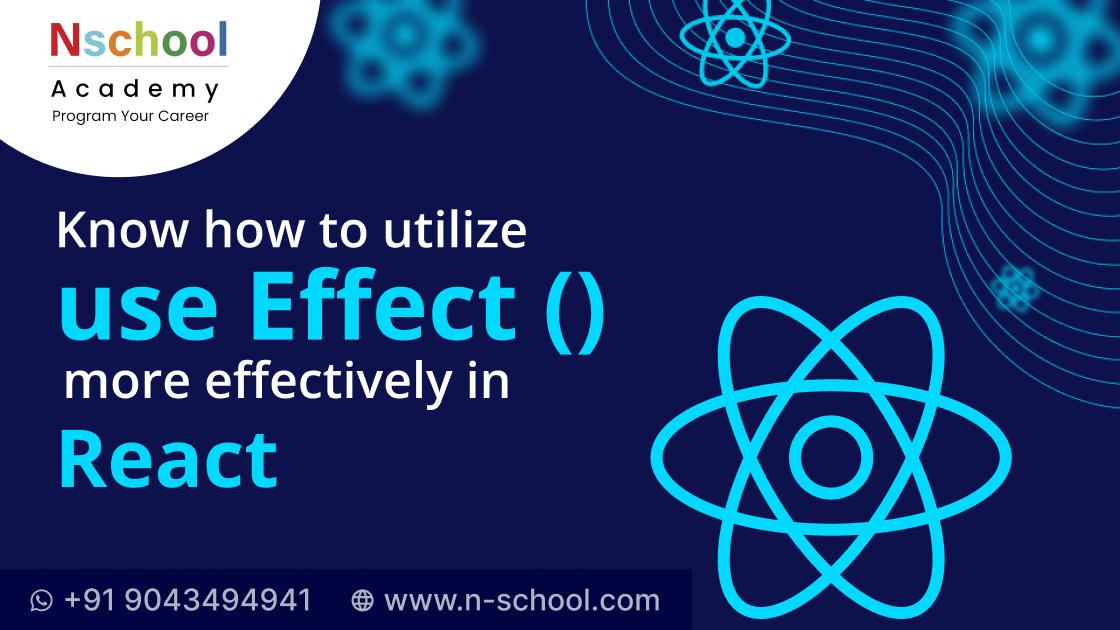
Know how to utilize useEffect more effectively in React?
The useEffect Hook was developed to mitigate the drawbacks of working with class-based components. DOM modifications, API data retrieval, subscription and timer creation, and other similar tasks can result in unexpected side effects. Since the render method is too quick to have a side impact, life cycle approaches are required to see the latter.
Changing the document’s title would be one simple way to contrast the present number visually. The clicked value is set to 0 when the rendering process begins. It is why the componentDidMount() method is called just once throughout the lifetime of a component. Then we construct a button that, when pressed, increments the value of the count state by one.
Because the document title is bound to the count number, each change to the title necessitates recreating the identical code in componentDidUpdate(). Whenever the state changes and the counter value has to be updated, using the componentDidupdate() method is required, which results in the same amount of code being reused each time.
componentDidMount(){
document.title = `you clicked ${this.state.count} times`;
}
Enabled Highly Dynamic Web Pages
React has inspired and made possible very interactive websites. The default suggested manner of writing React code does not, in experience, allow extremely dynamic sites. React employs a variety of ingenious ways internally to reduce the amount of expensive DOM operations needed to refresh the UI. A fast user interface may be achieved using React without much performance optimization in many apps. However, it can speed up your React app in many different ways. React creates and stores a local copy of the displayed user interface. Those components returned React elements are a part of this.
Since generating new DOM nodes and accessing existing ones might be time-consuming, React uses this format instead when possible.
React compares the newly returned element with the previously displayed one when a component’scomponent’s props or state changes to determine whether an actual DOM update is required. If they are different, React will alter the DOM accordingly. While React refreshes the affected DOM nodes, this process still takes time. In numerous cases, if the deceleration is noticeable, you can speed all of this up by overriding the lifecycle function Process.
The Rules of React Hooks in useEffect React
Before discussing more cases, we need to talk about how Hooks generally work. These are not unique to the useEffect React Hooks, but it is important to know where you can describe effects in your code.
- Hooks can only be called from the code at the top level of your React component.
- Hooks cannot be called from code inside another function body or loop.
- On the other hand, Custom React Hooks are special functions that can be called from the custom Hook’s top-level function. Rule number two is also true.
What are dependency array items?
It brings us to an important question: What should be in the dependency array? The React documentation says you must include all values from the component scope that change between renderings. Just what does this mean? All props, state variables, and context variables used inside of the useEffect callback function depend on the effect. Dependencies can also be Ref containers, which you get straight from useRef() and not the current value.
If, for some reason, you cannot move a function inside an effect, there are a few more options:
- Consider decoupling that feature from your component. Then, the function will never use any props or state, and it won’t be necessary to include it in the list of dependents.
- Instead of invoking the function within the effect, you may make the effect dependent on the function’s return value if it is a pure calculation that can be safely called during rendering.
- You may add a function to change dependencies as a last resort, but you must include its definition in the use clause. Callback React Hooks. As a result, it won’t be different every time it is rendered until the things it depends on also change.
- In effect, data fetching without the second dependents parameter might lead to this error. Without it, effects are triggered after each render, and resetting the state would do the same. If you use a variable in the dependency array, you risk creating an endless loop. Taking them out one by one will reveal which is the case. Removing a dependence, you rely on (or naively specifying []) is often the incorrect solution. As an alternative, you should attempt to address the underlying causes of the issue. Functions are one potential source of this issue, as are the related practices of enclosing, elevating, and wrapping them.
useEffect React after render
We know that useEffect() is used to make side effects in functional components, and it can also handle the componentDidMount(), componentDidUpdate(), componentWillUnmount() lifecycle methods of class-based components in functional components. The useEffect Hook allows you to perform side effects in functional components, and it is an immediate replacement for the componentDidMount(), componentDidUpdate(), and componentWillUnmount() processes.
What are the side effects in react?
Because they involve interaction with the “external world,” side effects cannot be predicted in advance. When we need to accomplish anything that is not directly related to our React components, we use a side effect. However, we cannot rely on the outcome of a side effect.
Consider the scenario when we request data (say, blog posts) from a broken server that provides us a 500 failure code instead of our post data. Except for the most basic uses, almost all apps need some kind of unintended consequence to function properly.
Common side effects in react include:
- Requesting information from a backend server using an API.
- Leverage the browser’s application programming interfaces (for direct document/window manipulation).
- Applying arbitrary timers like setTimeout and setInterval
To facilitate the execution of such non-essential tasks in otherwise pure React components, we have useEffect. For instance, we could modify the title meta element in the component to make the browser tab show the user’s name, but we should not.
function User({ name }) {
document.title = name;
// This is a side effect. Do not do this in the component body!
return <h1>{name}</h1>;
}
The rendering of our React component is disrupted if we execute a side effect immediately in the body of the component. It is important to keep the rendering process and the side effects apart. After our component has finished rendering, should we consider doing any necessary side effects? It is the result of using useEffect. Simply said, useEffect is a utility that allows us to communicate with the outside world without negatively impacting the visual appearance or overall performance of the component it is boarded.
How to fix common mistakes with useEffect
You need to know some small things about useEffect to avoid making mistakes. If you do not give useEffect any requirements and just give it a function, it will run after every draw. When you try to change the state in your useEffect React Hooks, this can cause trouble.
If you forget to give your dependencies correctly and set a piece of local state when the state changes, React will re-render the component by default. Without the dependents list, useEffect will run after every draw to have an endless loop.
function MyComponent() {
const [data, setData] = useState([])
useEffect(() => {
fetchData().then(myData => setData(myData))
// Error! useEffect runs after every render without the dependencies array, causing infinite loop
});
}
Cleanup function in useEffect
Sometimes we need to turn off our side affects. For example, if you use the setInterval function to make a countdown timer, that timer will not stop until you use the clearInterval function.
function Timer() {
const [time, setTime] = useState(0);
useEffect(() => {
setInterval(() => setTime(1), 1000);
// counts up 1 every second
// we need to stop using setInterval when component unmounts
}, []);
}
WebSockets accounts are another way to show this. When we finish a subscription, we must “turn it off.” It is what the cleanup method is for. If we use setInterval to change the state and do not clean up the side effect, the state will be killed along with the component when we stop using it, but the setInterval function will keep running.
Conclusion:
In functional components, useEffect(callback, dependencies) is the Hook that takes care of side effects. The callback input is a function that tells where to put the code for the side effect. Dependencies are a list of props or state values that your side-effect needs. useEffect(callback, dependencies) calls the callback after the first rendering (mounting) and on subsequent renderings if any value in dependencies has changed.
At Nschool Academy’s React JS Course in Coimbatore, you will learn how to master basic ideas like React JS Components, Frameworks, and Architecture with the help of Real-time React JS Developers. These are ideas like events, props, states, JSX, Redux, Hooks, and Performance optimization that a Professional React JS Developer needs to use.