- March 17, 2023
- nschool
- 0
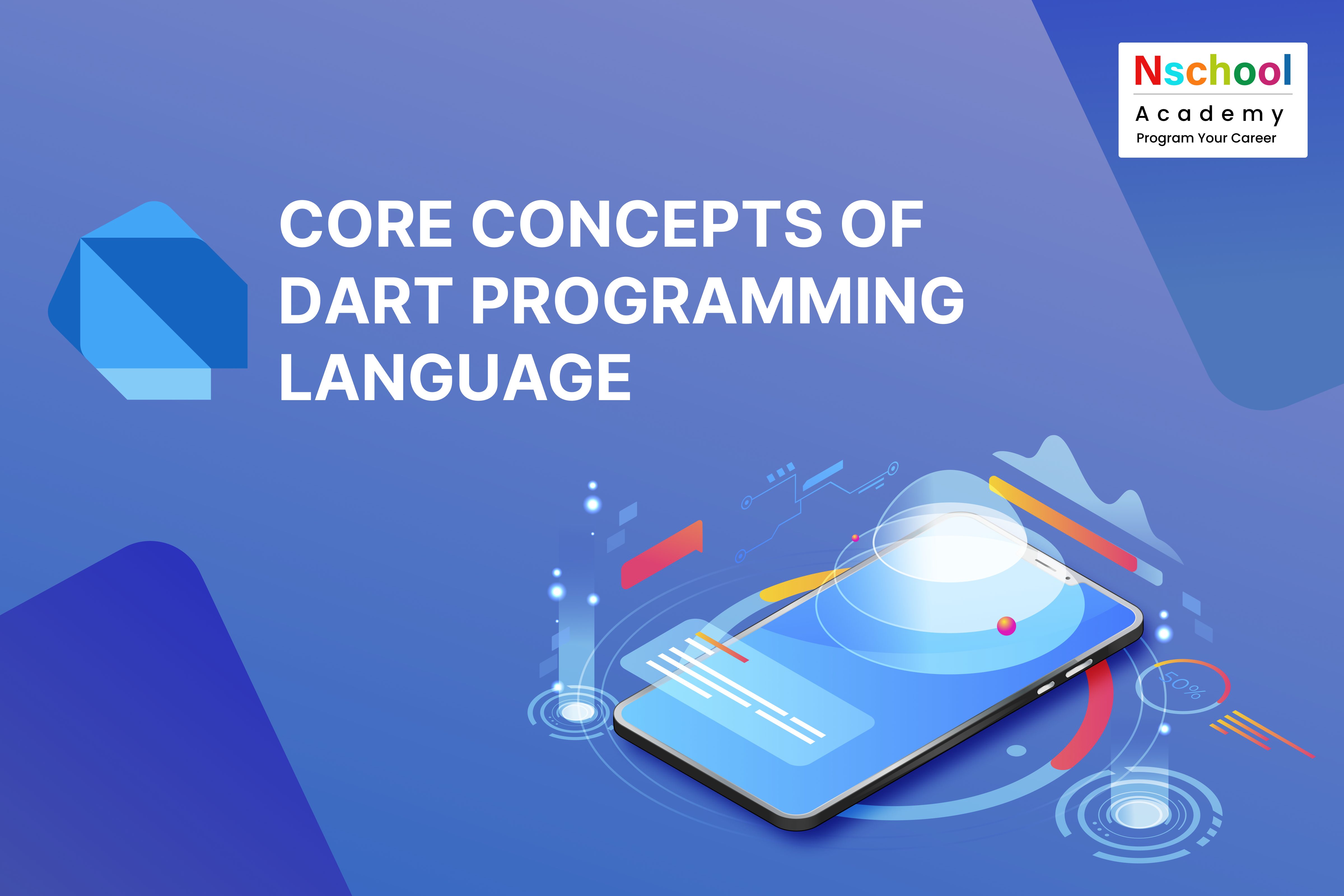
Know Core Concepts of Dart Programming Language Efficiently
Google developed Dart, a client-side multi-platform programming language, so that developers may rapidly build apps that can operate on various systems. Dart is a type-safe language. Hence, specifying the data type of any defined variables or modifiers is necessary. Dart’s built-in support for type inference means that, although types are mandatory, type requirements are not. Since we can’t be sure what information will be sent to us, we may create our variables as we choose. With the dynamic keyword and a comparison of types at runtime, we can deal with the unknown type as soon as it is discovered.
Flutter is an open-source UI framework for making native-feeling apps across mobile, web, and desktop platforms. Built on Dart Programming Language, it has Cupertino widgets and a design inspired by Google’s Material Design. Flutter allows designers to create attractive UIs that mimic native apps. Despite sharing the same codebase, its behavior remains unchanged across environments.
Dart Data Types
Dart is statically typed, meaning each var must be known when the code is compiled. The variable type cannot change when the program is running. Static typing is also used in C, Java, Swift, Dart Programming Language, and Kotlin.
On the other hand, languages like Python and JavaScript have dynamic typing. That means that the variables can hold different data when running the program. You don’t need to know the dart data types when you compile the code.
- int: Integers
- double: Floating-point numbers
- bool: Booleans
- String: Sequences of characters
- List
- Map
- Dynamic
Comments and Data Types
You’ll start by adding a variable assignment statement to the main. Variables store the information that your program will use. A variable is a container that stores a value in your computer’s memory. Each package is identified by its var name. Use the var keyword in Dart to represent a variable.
Comments
Comments in Dart look the same as in C and other languages: Text after / is a single-line comment, while text inside /*… */ is a multi-line comment block.
Operators
Dart has all the operators you’re used to from other languages like C, Swift, and Kotlin.
Some examples of Dart’s operators include:
- Arithmetic
- Equality
- Increment and decrement
- Comparison
- Logical
Control Flow
There are the Control Flows in Dart
- Conditionals
- While Loops
- Continue and Break
- For Loops
- Switch and case
- If Statements
Conditionals: The most basic form of control flow is deciding which parts of your code to run and which to skip over based on what happens as your program runs.
The if/else statement is how to handle conditions in a language. Suppose/else looks almost identical in Dart as in other C-like languages.
While Loops: You can use loops to repeat code several times or if certain conditions are met. You use while loops to repeat things based on needs. Dart has two different kinds of while loops: while and do-while. The difference is that the loop condition comes before the code block when using a time. The situation comes after in a do-while. So, do-while loops ensure the code block is run at least once.
Continue and Break: The keywords continue and break are used in loops and other places in Dart. What they do is:
- Continue: Jumps over the remaining code in a loop and goes straight to the next iteration.
- Break: Stops the loop and continues after its body.
For Loops: To loop a predetermined number of times in Dart, you use for loops. Initialization, a loop condition, and an action make up for loops. Again, they are similar to loops in other programming languages. A for-in loop, which iterates through a group of objects, is also available in Dart. You’ll find out more about these in the future.
Switch and case: In Dart, the == operator compares integer, string, or compile-time constants in switch statements. All objects being compared must be of the same class (and none of its subtypes), and the class can’t change the way == works. Switch statements work well with types from a list.
If and else: Dart helps if statements with optional else statements, as the next selection shows. Also, dwell conditional expressions.
if (isRaining()) {
you.bringRainCoat();
} else if (isSnowing()) {
you.wearJacket();
} else {
car.putTopDown();
}
Arithmetic Operators: Arithmetic operators function precisely as you’d expect them to. Try them out by adding a lot of operations to your DartPad:
Ex: print(20 / 2); // 10.0
Because DartPad formats the output to the console to show only the significant digits, the “20 / 2” result appears as “10” in the console. You will get 10.0 if you print the same statement using a Dart programme from the Dart SDK.
Comparison Operators
Dart uses the typical comparison operators:
- Less than (<)
- Greater than (>)
- Equal to (=>)
Nullability
When a variable wasn’t initialised in the past, Dart assigned it the value null, which meant that the variable contained no data. But as of Dart 2.12, it is no longer nullable by default, like Swift and Kotlin.
Also, Dart guarantees that a non-nullable type will never have a null value. It is known as proper null safety.
Dart Functions
Dart Functions allow you to combine several related lines of code into a single body. You then call the process, so you don’t have to write those lines of code in your Dart functions app repeatedly.
A function consists of the following elements:
- Return type
- Function name
- Parameter list in parentheses
- Function body enclosed in brackets
Access modifiers
Access/Visibility modifiers can be used to control how visible a class and its members are. The public, protected, and private keywords in a programming language like Java can be used to specify the visibility scope of a class’s data fields or methods.
Dart does not have a specific keyword for public, private, or protected data fields and methods. Dart gives us a way to handle this instead. All data fields are public by default, but you can make them private by putting an underscore “_” before the modifier you want to make private.
Objects and Classes in Dart
Object-oriented programming (OOP) is a method for developing complex software, and one of its core concepts is Classes. In computer science, a class is a concept that can be written down in syntax and is used to define how things behave and what they can do.
In computer science, a class is a plan, template, or agreement that details an object’s allowed data fields and action procedures. They specify how something may be utilized by providing a set of rules or guidelines. A class-level object’s instance cannot run a method or carry out an action that falls beyond the purview of the class’s specification.
Instantiating an object
A new instance of a class may be created in languages like Java by using the specialized “new” phrase. Yet, Dart was designed with Java and other statically typed languages in mind. For developers transitioning from a weakly typed language like JavaScript, removing the “new” keyword to create new objects in Dart 2 is a welcome change.
Inheritance
Like in real life, inheritance is a way for classes to get the properties and methods defined in another class. They allow for the creation of a new class from an existing class. The “superclass” is the class inherited from it, and the “sub-class” is inherited from it.
Encapsulation
Encapsulation is an OOP paradigm that lets us hide the values of a class’s data fields by preventing external operations from directly accessing them in a way that could reveal hidden implementation details. In a nutshell, encapsulation allows the programmer to hide and restrict access to data.
Abstraction
Class abstraction is the separation of how a class is used from how it is implemented. The internal implementation is hidden and out of sight. Dart’s internal methods, which we can use, are a good example of this. For example, the. Map () method of an array in Dart or the.toUpperCase() method of a String type. We don’t need to worry about the data fields it uses or how it does its job because they are hidden. We only need to know how the function works, what it needs as a parameter, and what it returns.
Polymorphism
Polymorphism is the ability of an object to assume different identities. Polymorphism is most frequently used in Dart when a subclass instance object is instantiated using a superclass reference type.
Named Parameters and Default Values
When there are multiple parameters, it can be hard to keep track of which is which. Named parameters to solve this problem in Dart are obtained by putting curly brackets {} around the parameter list.
Anonymous Functions
Dart supports first-class functions, which treat functions like any other data type. You can put them in variables, pass them as arguments, and get them back from other functions.
Remove the function name and return type to use these functions as values. This kind of function is known as an anonymous function because it has no name.
Conclusion:
This blog goes into great detail about how Dart Programming Language works. All of these ideas are important ones that we will need for job interviews and for making software in our daily lives. Professional developers in Coimbatore currently favour our Nschool Academies Flutter development courses with real-world industrial projects. The ideas are the same regardless of the programming language used. We can become real advanced-level software developers if we can master these ideas.